2. The above code will produce the following output . You may also want to check out all available functions/classes of the module google.cloud.storage , or try the search function . Here we will take an example image to show you how to do this. Convert A Blob To A File Using JavaScript March 10th, 2021. Specify the type of data contained within the specified field. the problem is : how can I convert this blob or brinary data to Mat format? Output. It's free to sign up and bid on jobs. POST. Use our online tool to encode an image to Base64 binary data. For example, if you have an image with id my-image . rsurathu: 0: 1,010: Aug-02-2020, 08:09 AM Last Post: rsurathu : Convert numpy array to image without . Hello friends, In this tutorial we saw how we can save text in a MySQL database: MySQLi. AngularJS . # Convert data into tuple format insert_blob_tuple = (image_id, Picture) . as I don't know the col and row of the image. We will start to read it using python opencv. Use a PreparedStatement and the setBlob () method, which writes the data into a BLOB and inserts the handle of the new BLOB into the SQL. To convert a string to a blob in JavaScript we are going to pass the string to a Blob constructor and return the string. Inserting images in a MySQL as a Blob using Python. Convert a String to a Blob object in JavaScript. You can also customize the same program for changing content type, content encoding, content md5 or cache control for the blobs. Second, create a cursor object using the connection object. Insert binary file in SQLite database with Python. After which, we traverse through the data in . First you need to set filterByColor = 1. Writing / Saving Images. Show. Next, create a function to convert digital data, i.e., images or any file, to binary data Convert to a Blob. thank you for your response, I am trying to create functions that allow the user to upload a picture and then save it in the sqlite3 database, so first in the def filedialogs function: the user will load an image, and in def convert_image_into_binary: it will convert the image to blob so I can add it in the database, but there is a problem with my method, so is there another method that will . Step 3: Convert the image to PDF using Python. In this quick tutorial we'll explore how to turn a Blob into a File. For the final step, you may use the template below in order to convert the image to PDF: from PIL import Image image_1 = Image.open (r'path where the image is stored\file name.png') im_1 = image_1.convert ('RGB') im_1.save (r'path where the pdf will be stored\new file name.pdf') For our example . The data type is used fo. For the same, we need to use the file.open () function to open the text file in "read" mode. Now we will convert this image to its base64 code using the below Python . And what's more important - we can use this encoding in "data-urls". const str2blob = txt => new Blob( [txt]); console.log(str2blob("Poopcode")) Search for jobs related to Dispay image blob field mysql using vbnet or hire on the world's largest freelancing marketplace with 21m+ jobs. At times, you may need to convert an image to a binary image. Hi, I get a block of data from socket, which is a image. Then we can set the src attribute of the img element to the base64 URL. Execute the SELECT query using cursor.execute () First, we import the base64 module into our Python script.. Once we have done so, we define a function, get_base64_encoded_image, that takes an image path as the parameter. Example of converting Image to base64 in Python import base64 with open ( "grayimage.png", "rb") as img_file: b64_string = base64.b64encode (img_file.read ()) print (b64_string) In my project folder, I have an image called grayimage.png. Convert jpeg to table. Convert Image to String. MySQL . import cv2 img = cv2.imread ('pic.jpg') cv2.imwrite ('img1.jpg', img) In this file, we will grab the image from MySQL database based on ID and . You can use the following code: import io from PIL import Image im = Image.open('test.jpg') im_resize = im.resize( (500, 500)) buf = io.BytesIO() im_resize.save(buf, format='JPEG') byte_im = buf.getvalue() In the above code, we save the im_resize Image object into BytesIO object buf. Delete. Project: loaner Author: google File: storage.py License: Apache License 2.0. Now click on the "Convert" button and then click on "To Image", enter the name that you want to save the file with, and from the type, box . At first, let's talk about the steps we gonna follow in this tutorial. Web Development . from fpdf import FPDF. Rather save the file/image to your server and only save the path to your data table. When we have the image path, we use the open function to get a file object to the image that we wish to encode in Base64.. The script below converts a fetch response into a Blob. Example of how to convert a base64 image in png format using python (the input file base64.txt used in the following example can be found here ): import base64 from PIL import Image from io import BytesIO f = open ('base64.txt', 'r') data = f.read () f.closed im = Image.open (BytesIO (base64.b64decode (data))) im.save ('image.png', 'PNG') We convert Blob to Base64 encoded string. 6 votes. Convert jpeg image to Byte array. Steps to Convert Colored Image to Binary Image. Set blobColor = 0 to select darker blobs, and blobColor = 255 for lighter blobs. Like other programming languages (e.g. Firstly, load the image as blob via XMLHttpRequest and use the FileReader API to convert it to a dataURL: Before getting started, let's install OpenCV. pysqlite - how to save images. Then we call response.blob to convert the binary data response to a blob . Found the solution public static string UploadImage(string imageData, ImageType imageType, int cardId) { string filename = GetFileName(imageType, cardId . Ubuntu . Read image using python opencv Import . Here we are displaying one image by using button and by using label. import mysql.connector import os import numpy as np from imgarray import save_array_img, load_array_img from os import fsync. Read datetime back from sqlite as a datetime in . Also at SQL developer I can previsualize the photos from table1, but not with table2. Videos you watch may be added to the TV's watch history and influence TV recommendations. # upload_blob_images_parallel.py # Python program to bulk upload jpg image files as blobs to azure storage # Uses ThreadPool for faster parallel uploads! Here is the code for converting an image to a string. From there, fire up a terminal and run the following command: $ python blob_from_images.py. If we can do so successfully, then we use base64.b64encode on the binary data of the image file. fetch ('./image . To do this we must make use of the regionprops_table function in Skimage. Using commit () method, We are saving the data. PHP. BLOB: Binary Large Object, it can store image, sound, video, pdf, video files There are several types: TINYBLOB: Stores up to 255 bytes. See the php documentation - file_put_contents The first terminal output is with respect to the first image found in the images folder where we apply the cv2.dnn.blobFromImage function: First Blob: (1, 3, 224, 224) The resulting beer glass image is displayed on the screen: printHeaders("Image upload example") 27 showFile() 28 printFooter() 29 Answer image is stored in database in binary format so once it comes to server using decode function to get it back to image 2 1 image.decode('base64') 2 this will convert your blob to image blob database html image python UserWarning: converting a masked element to nan imshow (tree_blobs, cmap = 'tab10'); We can see that the function identifies the different blobs in the image. A binary image is a 64bit encoded binary data that takes 33% more data than the original image. Inserting the data in the database in tuple format. python3 convert hex to binary 32 bit: Mkt: 3: 2,565: Aug-28-2020, 02:34 PM Last Post: Mkt : Convert xml to dict and group data and sum totals: kashcode: 5: 1,341: Aug-16-2020, 01:04 AM Last Post: scidam : How to convert a python code to binary? On clicking the 'Convert' button . We have the getImg function that makes a GET request to get an image from the imageUrl with fetch. Numpy array to SQL. Python, Sqlite3 - How to convert a list to a BLOB cell. Converting in Python is pretty straightforward, and the key part is using the "base64" module which provides standard data encoding an decoding. Put Text Data Into a Blob with Code Page: Select the Code Page to use when encoding the blob. img = result["IMAGE"][0].read() # reading the first BLOB result pre_img = io.BytesIO(img) Image.open(pre_img) This code works well, so the only problem is when I try to read images from table1 . Print the string. 1. To convert Base64 to PDF file in Python you need the base64.b64decode function and any function to write binary data into local files. It will return not only different Base64 values but also different images. Update. HTML . It is basically applied to images with less size for faster loading of the image. Import libraries for data handling. The first method we'll explore is converting a URL to an image using the OpenCV, NumPy, and the urllib libraries. Like other programming languages (e.g. First, establish the SQLite connection from Python. Once the upload is complete, the tool will convert the image to Base64 encoded binary data. If playback doesn't begin shortly, try restarting your device. blob-to-image.js This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. Convert String To Image Here To Convert It From String It Is Actually A Reverse Process Which Is Also Straight Forward Method First We Import Base64. String Field: Use the dropdown list to select the data field that will be converted to a Blob. For the "P" mode, this method translates pixels through the palette. Pandas how to find column contains a certain value Recommended way to install multiple Python versions on Ubuntu 20.04 Build super fast web scraper with Python x100 than BeautifulSoup How to convert a SQL query result to a Pandas DataFrame in Python How to write a Pandas DataFrame to a .csv file in Python Then We Open Our binary File Where We Dumped Our String. Perform Binarization on the Image. Syntax: Image.convert (mode=None, matrix=None, dither=None, palette=0, colors=256) Below I want to show you a basic example of how to do this, but before continuing I want to warn you that PDF files may contain malicious content that may jeopardize the security of users viewing such PDF files. import os. You can copy the encoded data by clicking in the output text areas. Be aware that this method may return different results for the same image depending on the browser and operating system. Store The Data That was Read From File Into A Variable. To retrieve, basically revers the sequence, use getBlob () on your resultset, then use ImageIO to read from . Then Open The File rb Mode Which Is R ead In B inary Mode. Sometimes you get a Blob object when instead you would like to have a File. I had the dataURL of the image that I converted to blob using the . To review, open the file in an editor that reveals hidden Unicode characters. If you'll be using the Base64 encoded data as an . Then we set pngImage.src to the URL that we'll get from the callback . Introduction - 1. It needs the base URL of an image to perform . This will return the base64 encoded data URI of the image. To read BLOB data from MySQL Table using Python, you need to follow these simple steps: - Install MySQL Connector Python using pip. properties = ['area','bbox','convex_area','bbox_area', 'major_axis_length', 'minor_axis_length', Then Close The File The next step now is to get the properties of each blob. Application Lifecycle > . Example 1. By Size : You can filter the blobs based on size by setting the parameters filterByArea = 1, and appropriate values for minArea and maxArea. setting minArea = 100 will filter out all the blobs that have less then 100 . Preliminary. Let's see the examples for converting the images into pdf file. Convert the Image to grayscale. Here is the code for converting an image to a string. Python-Image-Converter / app / convert.py / Jump to Code definitions message Function convert_raw Function convert_file Function ai_2_pdf Function image_not_exists Function checkExtension Function main Function # create a instance of fpdf. A data url has the form data: [<mediatype>] [;base64],<data>. In order to display the image/picture on the client you need to .NET class. Open up a new file, name it url_to_image.py , and let's get started: # import the necessary packages import numpy as np import urllib import cv2 # METHOD #1: OpenCV, NumPy, and urllib def url_to_image (url): # download the image . The first terminal output is with respect to the first image found in the images folder where we apply the cv2.dnn.blobFromImage function: First Blob: (1, 3, 224, 224) The resulting beer glass image is displayed on the screen: Run the following program to convert the content type of all files with extension .jpg to image/jpeg in Azure blob storage using Python SDK. (each time the image size if different) Mat image; image.create( rows, cols, CV_16UC1 ); memcpy . Steps for finding Centroid of a Blob in OpenCV. Java . a .NET solution for it. Image.convert () Returns a converted copy of this image. Converting an image to black and white involves two . Image below (from OpenCV Courses site) shows these steps. Create a SerialBlob from this byte array and then inject it into an INSERT or UPDATE sql statement. In this example, at first, we opened our file in 'rb' mode. The first solution that you may implement and probably the only idea that will occur to everybody, when you need to upload a Base64 image into the server, is to upload this file as a string and then convert it to a file in the server side.This implementation works like a charm, however there's another implementation that you may want to know if you don't want to make that your server does all . Retrieve the file: We can make an SQL query to retrieve the image. In other words, you need to convert a color image or greyscale image to black and white image. Convert to a Blob Field: Takes incoming data and converts it to a Blob. To find the center of the blob, we will perform the following steps:-. Use a PreparedStatement and the setBlob () method, which writes the data into a BLOB and inserts the handle of the new BLOB into the SQL. Storing BLOBs in a SQLite DB with Python/pysqlite. Step 3 Convert PDF files to JPG without Python. There are two conversion steps that have to be taken in order to obtain a blob file: Use Model Optimizer to produce OpenVINO IR representation (where IR stands for Intermediate Representation) Use Model Compiler to convert IR representation into MyriadX blob. Contribute to MaraGodeanu/Programe-Python development by creating an account on GitHub. Answer (1 of 7): [code]##### Using PIL from PIL import Image col = Image.open("test.jpg") #read image gray = col.convert('L') #conversion to gray scale bw = gray . To convert image from an Html page tag to a data URI using javascript, you first need to create a canvas element, set its width and height equal to that of the image, draw the image on it and finally call the toDataURL method on it. Step 3: Convert the image to PDF using Python. Click on the "File" and choose "Open", it will open a window for the user to locate the PDF files. Writing blob from SQLite to file using Python. Then we create an img element with document.createElement and assign it to pngImage . As a colored image has RGB layers in it and is more complex, convert it to its Grayscale form first. MySQL Blob ( Single )image in Tkinter. From there, fire up a terminal and run the following command: $ python blob_from_images.py. USAGE: python blob_samples_hello_world.py: Set the environment variables with your own values before running the sample: 1) AZURE_STORAGE_CONNECTION_STRING - the connection string to your storage account . Example 1: create a pdf from folder with multiple images. In this tutorial, we shall learn how to convert an image from color to black and white. Note that in this case, you have to specify the saving image . We can use such urls everywhere, on par with . Second, Establish MySQL database connection in Python. In this tutorial, you will learn how you can process images in Python using the OpenCV library. Set up a Threshold mark, pixels above the given mark will turn white, and below the mark will turn black. To write / save images in OpenCV using a function cv2.imwrite ()where the first parameter is the name of the new file that we will save and the second parameter is the source of the image itself. View Python questions; View Javascript questions; View Java questions; discussions forums. but not work. a bitmap to JPEG is a complex algorythm, you would need a e.g. Then we call document.body.appendChild to append the img element to the body. FileReader. Python pillow library also can read an image to numpy ndarray. The type of data is BLOB as describe(table) says in Oracle. Converting in Python is pretty straightforward, and the key part is using the "base64" module which provides standard data encoding an decoding. Rating property of a jpeg image. Java), we can also convert an image to a string representation in Python. The python and C++ codes used in this post are specifically for OpenCV 3.4.1. This sample demos basic blob operations like getting a blob client from container, uploading and downloading: a blob using the blob_client. As Olaf and Uri post, we can use the SQL Server binary and BLOBs data type to . 000webhost 0.- Now we are going to upload an image file and save it as a BLOB in MySQL. Choose any PDF files and open them in the PDFelement. read the image data. We have the svg variable with the SVG string. BLOB: Stores up to 65,535 bytes . 2. . Python . I tried below code. We will prepare an image which contains alpha chanel. 3. In this article we will see how we can save digital data like images, files, videos, songs etc into a MySQL database in the form of Blobs. This image is (width, height)=(180, 220), the backgroud of it is transparent. Get started by uploading your image by clicking the Upload image button. If mode is omitted, a mode is chosen so that all information in the image and the palette can be represented without a palette. If it's not JPEG: No way to do this in T-SQL, converting e.g. Advertisements. It's used to process images, videos, and even live streams, but in this tutorial, we will process images only as a first step. Then, define the SQLite INSERT Query. Find the center of the image after calculating the moments. As shown below: Step 3: Create "upload.php" file to store the image in the database. y_serial - warehouse compressed Python objects with SQLite. To display binary data as image in React, we can convert the image's binary data to a base64 URL. Convert Image to String. file = open('image.png','rb').read () file = base64.b64encode (file) args = ('100', 'Sample Name', file) query = 'INSERT INTO PROFILE VALUES (%s, %s, %s)' cursor.execute (query,args) mydb.commit () Now moving back to our MySQL database, we can see the inserted row. OpenCV is a free open source library used in real-time image processing. Create a SerialBlob from this byte array and then inject it into an INSERT or UPDATE sql statement. Having stored the script generated text file to PDF form, now let us convert the locally available text file to PDF form using the fpdf module. Below is the implementation: Python3 import cv2 img = cv2.imread ('ff.jpg', 2) ret, bw_img = cv2.threshold (img, 127, 255, cv2.THRESH_BINARY) Ajax . You can use the following code: import io from PIL import Image im = Image.open('test.jpg') im_resize = im.resize( (500, 500)) buf = io.BytesIO() im_resize.save(buf, format='JPEG') byte_im = buf.getvalue() In the above code, we save the im_resize Image object into BytesIO object buf. The resources on your server will be huge if you have many users adding data. How to convert Blob to File in JavaScript. echo "File uploaded successfully."; echo "File upload failed."; echo "Please select an image to upload."; Step 4: Create "display.php" file to get the image from the database. Web Design . How to convert to a binary image in PHP. Note that in this case, you have to specify the saving image . Open an image file. The image data is taken from Blob column of MySQL table. pdf = FPDF() pdf.set_auto_page_break(0) # use a folder that you created (here it's imgs) img_list = [x for x in os.listdir('imgs')] Then, Define the SELECT query to fetch BLOB column values from the database table. Output: 2. Twitter . For the final step, you may use the template below in order to convert the image to PDF: from PIL import Image image_1 = Image.open (r'path where the image is stored\file name.png') im_1 = image_1.convert ('RGB') im_1.save (r'path where the pdf will be stored\new file name.pdf') For our example . filename: my_image.jpg. converting tiff image into jpeg in windows 8 apps. Android . Then, why would you like to add large data (files, images etc) to a table field? To start, we call svgToPng with the svg string and the callback . I need to upload an image to NodeJS server to some directory. GET. SQL Server stores blobs in binary format. def insert_blob(self, path, contents, bucket_name=None): """Inserts a new json encoded Blob in the Cloud Storage bucket .
convert image to blob python
- Post author:
- Post published:June 16, 2022
- Post category:niall of the nine hostages genealogy
- Post comments:firmfit flooring topaz collection
convert image to blob pythonYou Might Also Like
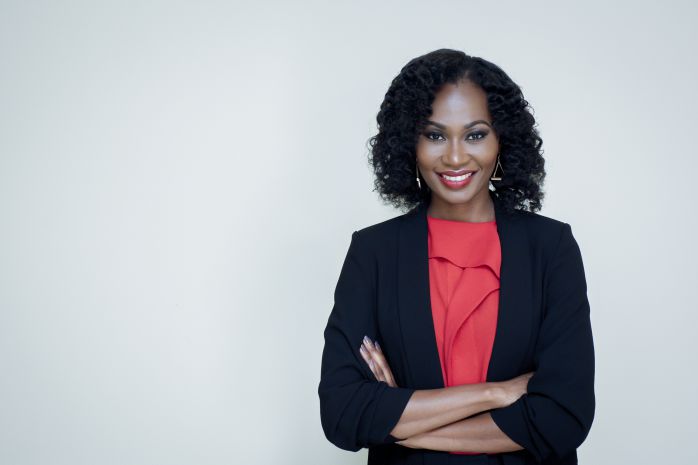
convert image to blob pythoninternet visio stencil

convert image to blob pythonhisense fridge error codes f1
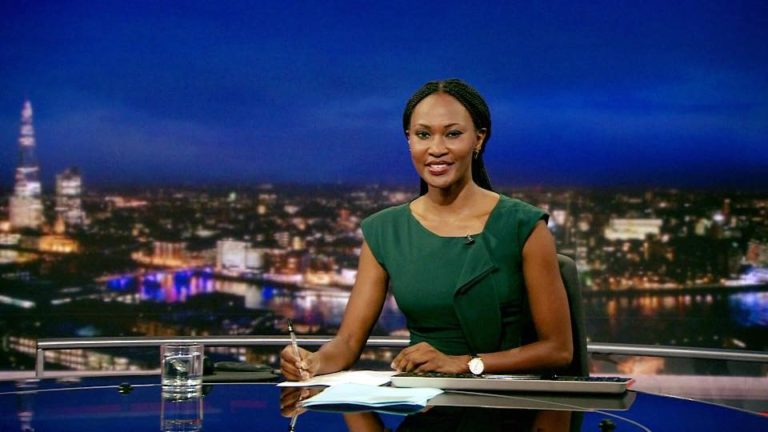